在窗体的状态栏上放置一个滚动的文本用来显示本地或者远程的一些有用信息是非常简单高效的。
.Net2.0下的状态栏是一个新类,名为StatusStrip,我们都知道它是个集合容器控件,默认情况下你可以向这个状态栏上添加标签、进度条和按钮等控件。不过,.Net真是一个不错的类库啊,我们可以利用框架提供的丰富的类进一步自定义状态栏来满足我们放置滚动条的功能。当我们在窗体上只放置了一个StatusStrip控件,并且仅添加了一个StatusLabel后,如下图:
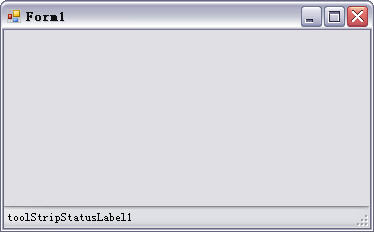
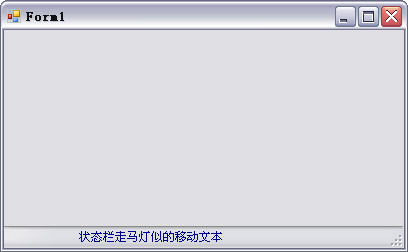
我们看一下Form1.Designer.cs文件的内容,尤其是那段微软所警告的那段代码:
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要
/// 使用代码编辑器修改此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.statusStrip1 = new System.Windows.Forms.StatusStrip();
this.toolStripStatusLabel1 = new System.Windows.Forms.ToolStripStatusLabel();
this.statusStrip1.SuspendLayout();
this.SuspendLayout();
//
// statusStrip1
//
this.statusStrip1.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.toolStripStatusLabel1});
this.statusStrip1.Location = new System.Drawing.Point(0, 176);
this.statusStrip1.Name = "statusStrip1";
this.statusStrip1.Size = new System.Drawing.Size(366, 22);
this.statusStrip1.TabIndex = 0;
this.statusStrip1.Text = "statusStrip1";
//
// toolStripStatusLabel1
//
this.toolStripStatusLabel1.Name = "toolStripStatusLabel1";
this.toolStripStatusLabel1.Size = new System.Drawing.Size(131, 17);
this.toolStripStatusLabel1.Text = "toolStripStatusLabel1";
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(366, 198);
this.Controls.Add(this.statusStrip1);
this.Name = "Form1";
this.Text = "Form1";
this.statusStrip1.ResumeLayout(false);
this.statusStrip1.PerformLayout();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
我们注意那段:this.toolStripStatusLabel1 = new System.Windows.Forms.ToolStripStatusLabel(); 原来,放置在StatusStrip上的Label控件需要实例化ToolStripStatusLabel类!这下就好办了,我们只需要自己动手创建一个继承自ToolStripStatusLabel的自定义类,然后实例化它并添加到statusStrip1.Items集合中。我们的新类取名为MovableTextToolStripStatusLabel,代码如下:
class MovableTextToolStripStatusLabel : ToolStripStatusLabel
{
private Timer updateTimer;
String drawString = "状态栏走马灯似的移动文本";
Font drawFont = new Font("宋体", 9);
SizeF stringSize = new SizeF();
SolidBrush drawBrush = new SolidBrush(Color.DarkBlue);
float x=0;
float y=2;
float width = 200;
float height = 17;
RectangleF drawRect = new RectangleF();
public MovableTextToolStripStatusLabel(): base()
{
this.updateTimer = new Timer();
this.updateTimer.Interval = 30;
this.updateTimer.Enabled = true;
this.updateTimer.Tick += delegate { this.OnTick(); };
drawRect.X = x;
drawRect.Y = y;
drawRect.Width = width;
drawRect.Height = height;
x = 0 - stringSize.Width;
}
private void OnTick()
{
x++;
if (x > this.width + stringSize.Width)
x = 0 - stringSize.Width;
drawRect.X = x;
this.Invalidate();
}
protected override void OnPaint(PaintEventArgs e)
{
stringSize = e.Graphics.MeasureString(drawString, drawFont);
e.Graphics.DrawString(drawString, drawFont, drawBrush, drawRect);
base.OnPaint(e);
}
}
我们在MovableTextToolStripStatusLabel内部创建一个计数器用来控制文本移动速度和位置,利用Graphics.MeasureString计算好滚动文本占用的矩形框大小,然后在计时器的OnTick事件中Invalidate我们的这个自定义ToolStripStatusLabel表面,以便激活OnPaint事件,说到头还是离不开绘制,再利用Graphics.DrawString将文本显示出来。
接着,将Form1.Designer.cs文件中的关于ToolStripStatusLabel类替换成MovableTextToolStripStatusLabel类,代码如下:
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要
/// 使用代码编辑器修改此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.statusStrip1 = new System.Windows.Forms.StatusStrip();
this.movabletextToolStripStatusLabel = new WindowsApplication1.MovableTextToolStripStatusLabel();
this.statusStrip1.SuspendLayout();
this.SuspendLayout();
//
// statusStrip1
//
this.statusStrip1.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.movabletextToolStripStatusLabel});
this.statusStrip1.Location = new System.Drawing.Point(0, 196);
this.statusStrip1.Name = "statusStrip1";
this.statusStrip1.Size = new System.Drawing.Size(400, 22);
this.statusStrip1.TabIndex = 0;
this.statusStrip1.Text = "statusStrip1";
//
// movabletextToolStripStatusLabel
//
this.movabletextToolStripStatusLabel.AutoSize = false;
this.movabletextToolStripStatusLabel.Name = "movabletextToolStripStatusLabel";
this.movabletextToolStripStatusLabel.Size = new System.Drawing.Size(300, 17);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(400, 218);
this.Controls.Add(this.statusStrip1);
this.Name = "Form1";
this.Text = "Form1";
this.statusStrip1.ResumeLayout(false);
this.statusStrip1.PerformLayout();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
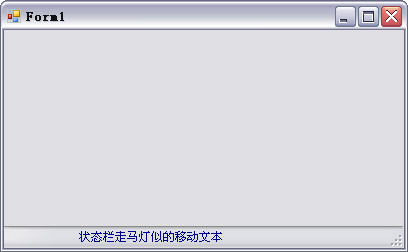
一个内嵌走马灯似的滚动文本的状态栏就实现了。